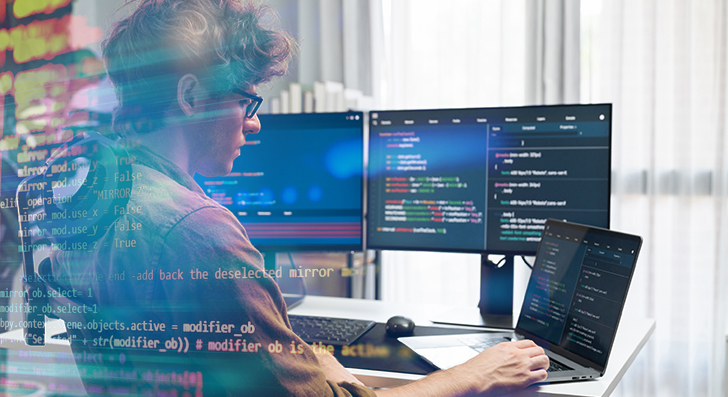
Scalability signifies your software can cope with progress—a lot more users, extra knowledge, and a lot more site visitors—with out breaking. Like a developer, building with scalability in your mind saves time and worry later on. Here’s a transparent and sensible guideline that can assist you start off by Gustavo Woltmann.
Style for Scalability from the beginning
Scalability is not a little something you bolt on later on—it ought to be element of your prepare from the beginning. Lots of programs are unsuccessful after they mature quickly because the initial structure can’t cope with the extra load. Being a developer, you need to Consider early regarding how your method will behave stressed.
Get started by developing your architecture to generally be versatile. Stay clear of monolithic codebases in which anything is tightly connected. As an alternative, use modular structure or microservices. These patterns break your app into scaled-down, impartial sections. Each module or support can scale on its own devoid of influencing the whole program.
Also, contemplate your databases from day 1. Will it need to have to take care of 1,000,000 buyers or just a hundred? Select the appropriate sort—relational or NoSQL—based upon how your details will develop. Prepare for sharding, indexing, and backups early, Even when you don’t have to have them yet.
Yet another significant issue is to prevent hardcoding assumptions. Don’t produce code that only is effective under existing problems. Give thought to what would happen In case your user base doubled tomorrow. Would your application crash? Would the databases decelerate?
Use layout designs that assist scaling, like concept queues or function-pushed programs. These support your application tackle extra requests without the need of having overloaded.
When you Construct with scalability in your mind, you're not just preparing for success—you might be lowering long term headaches. A well-prepared process is simpler to maintain, adapt, and grow. It’s improved to get ready early than to rebuild afterwards.
Use the appropriate Database
Choosing the ideal databases is actually a important part of making scalable apps. Not all databases are developed precisely the same, and using the Completely wrong one can slow you down or simply lead to failures as your app grows.
Get started by knowledge your info. Could it be extremely structured, like rows inside of a table? If yes, a relational databases like PostgreSQL or MySQL is a good healthy. These are generally powerful with interactions, transactions, and consistency. They also aid scaling tactics like read replicas, indexing, and partitioning to manage much more targeted visitors and info.
If your knowledge is a lot more versatile—like user exercise logs, item catalogs, or paperwork—consider a NoSQL selection like MongoDB, Cassandra, or DynamoDB. NoSQL databases are better at dealing with substantial volumes of unstructured or semi-structured information and might scale horizontally more conveniently.
Also, think about your examine and write designs. Will you be performing a great deal of reads with much less writes? Use caching and read replicas. Have you been dealing with a major publish load? Take a look at databases that may take care of superior write throughput, and even celebration-centered data storage methods like Apache Kafka (for short term data streams).
It’s also wise to Consider in advance. You might not have to have advanced scaling attributes now, but selecting a database that supports them signifies you gained’t will need to switch later.
Use indexing to speed up queries. Stay away from needless joins. Normalize or denormalize your knowledge determined by your entry styles. And generally watch databases general performance as you develop.
In brief, the proper database depends upon your app’s structure, velocity requires, And exactly how you hope it to improve. Consider time to pick sensibly—it’ll help you save loads of issues later on.
Enhance Code and Queries
Quickly code is key to scalability. As your application grows, each individual smaller hold off provides up. Badly composed code or unoptimized queries can slow down performance and overload your procedure. That’s why it’s essential to Create productive logic from the start.
Get started by producing clear, basic code. Keep away from repeating logic and remove just about anything unwanted. Don’t select the most complex Option if an easy 1 is effective. Maintain your functions small, targeted, and easy to check. Use profiling resources to uncover bottlenecks—spots exactly where your code usually takes way too lengthy to operate or makes use of too much memory.
Following, have a look at your database queries. These typically slow factors down greater than the code alone. Make certain Just about every query only asks for the information you actually need to have. Avoid Find *, which fetches every little thing, and in its place pick unique fields. Use indexes to speed up lookups. And keep away from doing too many joins, In particular across huge tables.
For those who recognize the same info remaining requested over and over, use caching. Retail outlet the results temporarily employing applications like Redis or Memcached so that you don’t really need to repeat highly-priced operations.
Also, batch your database functions after you can. Rather than updating a row one by one, update them in teams. This cuts down on overhead and would make your application more effective.
Remember to examination with substantial datasets. Code and queries that do the job good with 100 documents could possibly crash once they have to deal with 1 million.
In a nutshell, scalable apps are rapidly applications. Maintain your code restricted, your queries lean, and use caching when wanted. These techniques assistance your software continue to be easy and responsive, even as the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to deal with more users and much more visitors. If every little thing goes by means of a single server, it's going to swiftly turn into a bottleneck. That’s wherever load balancing and caching can be found in. These two resources assist keep your application speedy, secure, and scalable.
Load balancing spreads incoming targeted traffic across numerous servers. Rather than 1 server doing each of the function, the load balancer routes users to distinctive servers based upon availability. What this means is no single server gets overloaded. If just one server goes down, the load balancer can ship traffic to the others. Applications like Nginx, HAProxy, or cloud-dependent answers from AWS and Google Cloud make this easy to build.
Caching is about storing details briefly so it can be reused immediately. When end users request a similar data once more—like an item website page or perhaps a profile—you don’t really need to fetch it through the database anytime. You may serve it within the cache.
There are 2 popular forms of caching:
1. Server-aspect caching (like Redis or Memcached) stores knowledge in memory for quick entry.
2. Customer-side caching (like browser caching or CDN caching) merchants static files near to the user.
Caching lessens database load, enhances velocity, and helps make your application much more successful.
Use caching for things that don’t adjust often. And often be certain your cache is up to date when facts does alter.
In short, load balancing and caching are basic but powerful equipment. Alongside one another, they help your app cope with more consumers, keep fast, and Recuperate from challenges. If you propose to develop, you may need both of those.
Use Cloud and Container Equipment
To make scalable applications, you will need instruments that permit your application grow very easily. That’s the place cloud platforms and containers are available. They offer you flexibility, decrease setup time, and make scaling Considerably smoother.
Cloud platforms like Amazon World-wide-web Products and services (AWS), Google Cloud System (GCP), and Microsoft Azure Allow you to lease servers and expert services as you would like them. You don’t really have to buy hardware or guess long term capacity. When site visitors will increase, it is possible to incorporate additional methods with just a couple clicks or mechanically working with vehicle-scaling. When traffic drops, you can scale down to save money.
These platforms also offer services like managed databases, storage, load balancing, and stability applications. You could deal with making your application as an alternative to controlling infrastructure.
Containers are Yet another important tool. A container offers your application and every little thing click here it has to run—code, libraries, settings—into one device. This causes it to be simple to maneuver your application among environments, from your notebook to your cloud, with no surprises. Docker is the most popular Software for this.
Whenever your app uses many containers, equipment like Kubernetes assist you to manage them. Kubernetes handles deployment, scaling, and Restoration. If one particular component of your application crashes, it restarts it immediately.
Containers also enable it to be very easy to separate portions of your app into products and services. It is possible to update or scale components independently, which happens to be great for general performance and dependability.
In short, working with cloud and container resources means you may scale quick, deploy quickly, and recover promptly when issues transpire. If you would like your application to grow with no limits, commence applying these resources early. They help save time, decrease chance, and help you remain centered on building, not repairing.
Observe Every little thing
When you don’t monitor your application, you gained’t know when matters go Incorrect. Monitoring will help the thing is how your application is carrying out, place difficulties early, and make better decisions as your app grows. It’s a essential Element of building scalable techniques.
Start off by tracking fundamental metrics like CPU utilization, memory, disk Room, and reaction time. These show you how your servers and products and services are doing. Resources like Prometheus, Grafana, Datadog, or New Relic can assist you accumulate and visualize this facts.
Don’t just observe your servers—monitor your app too. Keep an eye on how long it will take for consumers to load webpages, how often mistakes take place, and the place they manifest. Logging applications like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can help you see what’s going on within your code.
Arrange alerts for vital complications. Such as, In the event your reaction time goes earlier mentioned a Restrict or possibly a support goes down, you ought to get notified right away. This aids you repair issues fast, normally right before people even observe.
Monitoring can also be beneficial whenever you make changes. For those who deploy a different attribute and see a spike in faults or slowdowns, you may roll it back again prior to it results in authentic injury.
As your app grows, targeted visitors and knowledge improve. Without the need of checking, you’ll miss indications of problems till it’s much too late. But with the best tools in position, you stay in control.
Briefly, monitoring can help you keep your application dependable and scalable. It’s not pretty much spotting failures—it’s about being familiar with your program and ensuring that it works perfectly, even under pressure.
Remaining Ideas
Scalability isn’t only for large corporations. Even little applications require a robust Basis. By developing diligently, optimizing properly, and utilizing the right instruments, you can Establish apps that increase smoothly without having breaking stressed. Start modest, Imagine large, and Make smart.